Daemonjax
First time out of the vault
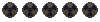
I thought it would be simple matter to just call obj_set_light_level(dude_obj,0,0); at the appropriate time(s) (as mentioned on the sfall request page), but it doesn't work properly all the time and I don't have the information to figure it out.
http://falloutmods.wikia.com/wiki/Sfall_Requests
Here's what I got so far:
It works when moving the flare from the left hand to the inventory, but not when moving it from the right hand when the left hand is the one actively displayed. It probably has nothing to do with right or left hands, and it just affects whatever flare is in the offhand.
Important note: display_msg("neither hand still holds a flare"); fires in both cases during testing, so I know obj_set_light_level(dude_obj,0,0); is also firing in both cases (left or right hand), so the code itself is not the problem. Plus the fact that the light flickers off and back on -- but the mainhand works perfectly, which is weird.
Either obj_set_light_level itself is failing, or something else is turning the light back on after obj_set_light_level turns the light off. I'm thinking the latter, since I can see the light flicker off and then back on.
So, I started looking into exactly how flares work, and I can't find any information (using RP 2.3.3). And sfall (of course).
I've grepped all my sources and headers looking for clues, and I got nothing much mentioning flares (besides one #define for item188 which was a dead end). I've also looked at killap's global scripts... nothing. I've also used the forum search feature, expecting this to have come up in some shape or form, but nothing. It's like flares are a black box.
I know there's additional work I'll need to do after map transitions, but I want to tackle this offhand problem first.
EDIT: It gets weirder, but simpler in a way:
If I put/take anything into/out of the dude's active hand after turning the light off, the light comes back on. How does that happen? It's like there's a competing hook script, but I can't find it.
I'll add another check to the above code for anything going into the hands and see if that does it.
EDIT2: I can't find a way to turn the light off which is turned on after any object is added to the dude's hands (obj_set_light_level(dude_obj,0,0) fires but is unsuccessful). Why does it do that? Something somewhere is turning it on, but where? Is it built into the engine? Maybe if I use the mouseclick hook...
Any information would be helpful.
EDIT3: Using the mouseclick hook for this works well enough. That should work out fine, and I could do something kooky like have the light turn on everytime you open your inventory as if you're using the pipboy as a light to rummage through your stuff. May as well make a toggle button for it, too.
This code in obj_dude prevents the light from coming back on for no reason after map transitions:
EDIT: Actually, obj_pid(inven_ptr(dude_obj, 1)) doesn't work as expected in any script I've tried it in. I imagine obj_pid works as expected since I use it a lot, but I'm not sure what's going on with inven_ptr. I get bogus pids for stuff in both hands. Dunno. It's just a #define, so the #define could be messed up. I can't enable flares lit in hands without it working.
My #define's for it look right:
#define INVEN_CMD_INDEX_PTR (13)
#define inven_ptr(WHO,WHERE) inven_cmds(WHO,INVEN_CMD_INDEX_PTR,WHERE)
No idea what could be up with inven_ptr returning bogus objects. How else would I get what's in the players' hands? Maybe my slot numbers are wrong...
Did some digging and this should work:
firstHand := obj_pid(critter_inven_obj(dude_obj, INVEN_TYPE_LEFT_HAND));
secondHand := obj_pid(critter_inven_obj(dude_obj, INVEN_TYPE_RIGHT_HAND));
EDIT: Whew. I'm done. I had to use quite a few hook scripts to get it working really well, but it's done. In case anyone else is interested (just the pertinent code I added to these files):
obj_dude.ssl
hs_mouseclick (this is resolution dependent)
hs_combatdamage
hs_keypress
Ended up not using hs_inventorymove at all. I wonder if there was an easier way to do all this. Does light make it harder to sneak? If not I should add that in. And I still don't know how flares work.
EDIT: It would probably be better to use a global instead of exporting/importing invLightIsOn because I don't know what would happen if I used the keyboard before clicking the mouse after the game loads. Probably something bad.
http://falloutmods.wikia.com/wiki/Sfall_Requests
- Can we add an option to disable default light source "attached" to the player? aka the "Egg". Which would mean no highlight on tiles/walls/scenery/whatever in night, the scenery wont glow. In other words: use flares, or walk in dark. (use obj_set_light_level(dude_obj,0,0) in obj_dude.int or global script)
Here's what I got so far:
Code:
/*
hs_inventorymove.int
Runs before moving items between inventory slots in dude interface. You can override the action.
What you can NOT do with this hook:
- force moving items to inapropriate slots (like gun in armor slot)
- block picking up items
What you can do:
- restrict player from using specific weapons or armors
- add AP costs for all inventory movement including reloading
- apply or remove some special scripted effects depending on PC's armor
int arg1 - Target slot (0 - main backpack, 1 - left hand, 2 - right hand, 3 - armor slot, 4 - weapon, when reloading it by dropping ammo)
Item arg2 - Item being moved
Item arg3 - Item being replaced or weapon being reloaded (can be 0)
int ret1 - Override setting (-1 - use engine handler, any other value - prevent relocation of item/reloading weapon)
*/
#include ".\headers\define.h"
#define UNLIT_FLARE (79)
#define LIT_FLARE (205)
procedure start;
procedure start begin
variable slot, item, replaced, firstHand, secondHand;
if not (init_hook) then begin
slot := get_sfall_arg;
item := get_sfall_arg;
replaced := get_sfall_arg;
//display_msg("slot: " + slot + " item: " + item);
if (slot == 0 and (obj_pid(item) == UNLIT_FLARE or obj_pid(item) == LIT_FLARE)) then begin
//display_msg("flare moved to inventory");
firstHand := obj_pid(inven_ptr(dude_obj, 1));
secondHand := obj_pid(inven_ptr(dude_obj, 2));
// if neither hand still holds a flare
if (firstHand == UNLIT_FLARE or secondHand == UNLIT_FLARE
or firstHand == LIT_FLARE or secondHand == LIT_FLARE) then begin
end else begin
display_msg("neither hand still holds a flare");
obj_set_light_level(dude_obj,0,0); // turn off the dude's light
end
end
end
end
It works when moving the flare from the left hand to the inventory, but not when moving it from the right hand when the left hand is the one actively displayed. It probably has nothing to do with right or left hands, and it just affects whatever flare is in the offhand.
Important note: display_msg("neither hand still holds a flare"); fires in both cases during testing, so I know obj_set_light_level(dude_obj,0,0); is also firing in both cases (left or right hand), so the code itself is not the problem. Plus the fact that the light flickers off and back on -- but the mainhand works perfectly, which is weird.
Either obj_set_light_level itself is failing, or something else is turning the light back on after obj_set_light_level turns the light off. I'm thinking the latter, since I can see the light flicker off and then back on.
So, I started looking into exactly how flares work, and I can't find any information (using RP 2.3.3). And sfall (of course).
I've grepped all my sources and headers looking for clues, and I got nothing much mentioning flares (besides one #define for item188 which was a dead end). I've also looked at killap's global scripts... nothing. I've also used the forum search feature, expecting this to have come up in some shape or form, but nothing. It's like flares are a black box.
I know there's additional work I'll need to do after map transitions, but I want to tackle this offhand problem first.
EDIT: It gets weirder, but simpler in a way:
If I put/take anything into/out of the dude's active hand after turning the light off, the light comes back on. How does that happen? It's like there's a competing hook script, but I can't find it.
I'll add another check to the above code for anything going into the hands and see if that does it.
EDIT2: I can't find a way to turn the light off which is turned on after any object is added to the dude's hands (obj_set_light_level(dude_obj,0,0) fires but is unsuccessful). Why does it do that? Something somewhere is turning it on, but where? Is it built into the engine? Maybe if I use the mouseclick hook...
Any information would be helpful.
EDIT3: Using the mouseclick hook for this works well enough. That should work out fine, and I could do something kooky like have the light turn on everytime you open your inventory as if you're using the pipboy as a light to rummage through your stuff. May as well make a toggle button for it, too.
This code in obj_dude prevents the light from coming back on for no reason after map transitions:
Code:
procedure map_enter_p_proc begin
// dj
variable firstHand := obj_pid(inven_ptr(dude_obj, 1));
variable secondHand := obj_pid(inven_ptr(dude_obj, 2));
if (firstHand == 79 or secondHand == 79
or firstHand == 205 or secondHand == 205) then begin
end else begin
//display_msg("neither hand is holding a flare so turn off light");
obj_set_light_level(dude_obj,0,0);
end
EDIT: Actually, obj_pid(inven_ptr(dude_obj, 1)) doesn't work as expected in any script I've tried it in. I imagine obj_pid works as expected since I use it a lot, but I'm not sure what's going on with inven_ptr. I get bogus pids for stuff in both hands. Dunno. It's just a #define, so the #define could be messed up. I can't enable flares lit in hands without it working.
My #define's for it look right:
#define INVEN_CMD_INDEX_PTR (13)
#define inven_ptr(WHO,WHERE) inven_cmds(WHO,INVEN_CMD_INDEX_PTR,WHERE)
No idea what could be up with inven_ptr returning bogus objects. How else would I get what's in the players' hands? Maybe my slot numbers are wrong...
Did some digging and this should work:
firstHand := obj_pid(critter_inven_obj(dude_obj, INVEN_TYPE_LEFT_HAND));
secondHand := obj_pid(critter_inven_obj(dude_obj, INVEN_TYPE_RIGHT_HAND));
EDIT: Whew. I'm done. I had to use quite a few hook scripts to get it working really well, but it's done. In case anyone else is interested (just the pertinent code I added to these files):
obj_dude.ssl
Code:
procedure map_enter_p_proc begin
//turns off light on map transition if not holding flares
variable firstHand;
variable secondHand;
firstHand := obj_pid(critter_inven_obj(dude_obj, INVEN_TYPE_LEFT_HAND));
secondHand := obj_pid(critter_inven_obj(dude_obj, INVEN_TYPE_RIGHT_HAND));
if (firstHand == 79 or secondHand == 79
or firstHand == 205 or secondHand == 205) then begin
end else begin
//display_msg("neither hand still holds a flare so turn off light");
obj_set_light_level(dude_obj,0,0);
end
hs_mouseclick (this is resolution dependent)
Code:
//1920x1080 = 960x540(internal)
//#define INV_UPPER_LEFT_Xa (371)
//#define INV_LOWER_RIGHT_Xa (402)
//#define INV_UPPER_LEFT_Ya (481)
//#define INV_LOWER_RIGHT_Ya (501)
//#define INVDONE_UPPER_LEFT_Xa (668)
//#define INVDONE_LOWER_RIGHT_Xa (681)
//#define INVDONE_UPPER_LEFT_Ya (361)
//#define INVDONE_LOWER_RIGHT_Ya (376)
//1706x960 = 853x480(internal)
#define INV_UPPER_LEFT_X (320)
#define INV_LOWER_RIGHT_X (348)
#define INV_UPPER_LEFT_Y (421)
#define INV_LOWER_RIGHT_Y (441)
#define INVDONE_UPPER_LEFT_X (614)
#define INVDONE_LOWER_RIGHT_X (628)
#define INVDONE_UPPER_LEFT_Y (331)
#define INVDONE_LOWER_RIGHT_Y (346)
#define UNLIT_FLARE (79)
#define LIT_FLARE (205)
variable invGetNext := 0;
variable invDoneGetNext := 0
export variable invLightIsOn := 0;
// turn off light when inventory closed
if (invLightIsOn and window == 1) then begin
x := get_mouse_x;
y := get_mouse_y;
if (invDoneGetNext and (event == UP) and (button == LEFT)) then begin
invDoneGetNext := 0;
if ((x >= INVDONE_UPPER_LEFT_X) and (x <= INVDONE_LOWER_RIGHT_X)
and (y >= INVDONE_UPPER_LEFT_Y) and (y <= INVDONE_LOWER_RIGHT_Y)) then begin
//closing inventory
firstHand := obj_pid(critter_inven_obj(dude_obj, INVEN_TYPE_LEFT_HAND));
secondHand := obj_pid(critter_inven_obj(dude_obj, INVEN_TYPE_RIGHT_HAND));
//display_msg("firstHand:" + firstHand + " secondHand:" + secondHand);
if (firstHand == UNLIT_FLARE or secondHand == UNLIT_FLARE
or firstHand == LIT_FLARE or secondHand == LIT_FLARE) then begin
end else begin
//display_msg("neither hand still holds a flare so turn off light");
obj_set_light_level(dude_obj,0,0);
end
invLightIsOn := 0; //set this to 0 regardless
end
end else if ((event == DOWN) and (button == LEFT)) then begin
invDoneGetNext := 0;
if ((x >= INVDONE_UPPER_LEFT_X) and (x <= INVDONE_LOWER_RIGHT_X)
and (y >= INVDONE_UPPER_LEFT_Y) and (y <= INVDONE_LOWER_RIGHT_Y)) then begin
invDoneGetNext := 1;
end
end
end else begin
invDoneGetNext := 0;
end
//turn on light when inventory opened
if (window == 3) then begin
x := get_mouse_x;
y := get_mouse_y;
if (invGetNext and (event == UP) and (button == LEFT)) then begin
invGetNext := 0;
if ((x >= INV_UPPER_LEFT_X) and (x <= INV_LOWER_RIGHT_X)
and (y >= INV_UPPER_LEFT_Y) and (y <= INV_LOWER_RIGHT_Y)) then begin
//definitely opening inventory so turn light on
invLightIsOn := 1;
obj_set_light_level(dude_obj,100,4);
end
end else if ((event == DOWN) and (button == LEFT)) then begin
invGetNext := 0;
if ((x >= INV_UPPER_LEFT_X) and (x <= INV_LOWER_RIGHT_X)
and (y >= INV_UPPER_LEFT_Y) and (y <= INV_LOWER_RIGHT_Y)) then begin
invGetNext := 1;
end
end
end else begin
invGetNext := 0;
end
hs_combatdamage
Code:
// dj this block is just for turning off the egg light when out of flares
weaponPid := obj_pid(weapon);
if (weaponPid == UNLIT_FLARE or weaponPid == LIT_FLARE) then begin
if (obj_is_carrying_obj(dude_obj, weaponPid) > 0) then begin // needs the > 0 part
end else begin
//display_msg("out of flares of thrown type in inventory so turn off light");
obj_set_light_level(dude_obj,0,0);
end
end
hs_keypress
Code:
import variable invLightIsOn;
if (keyDX == DIK_I) then begin
invLightIsOn := 1;
obj_set_light_level(dude_obj,100,4);
end else
if (keyDX == DIK_ESCAPE) then begin
firstHand := obj_pid(critter_inven_obj(dude_obj, INVEN_TYPE_LEFT_HAND));
secondHand := obj_pid(critter_inven_obj(dude_obj, INVEN_TYPE_RIGHT_HAND));
//display_msg("firstHand:" + firstHand + " secondHand:" + secondHand);
if (firstHand == UNLIT_FLARE or secondHand == UNLIT_FLARE
or firstHand == LIT_FLARE or secondHand == LIT_FLARE) then begin
end else begin
//display_msg("neither hand still holds a flare so turn off light");
obj_set_light_level(dude_obj,0,0);
end
invLightIsOn := 0; //set this to 0 regardless
end
Ended up not using hs_inventorymove at all. I wonder if there was an easier way to do all this. Does light make it harder to sneak? If not I should add that in. And I still don't know how flares work.

EDIT: It would probably be better to use a global instead of exporting/importing invLightIsOn because I don't know what would happen if I used the keyboard before clicking the mouse after the game loads. Probably something bad.
Last edited: