QuantumApprentice
Where'd That 6th Toe Come From?
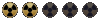
There are a whole bunch of Nicole's dialogue lines I want to play from a basic debug menu I created, but instead of creating a unique procedure for every single line of dialogue, is it possible to have a generic response procedure that you just call from the base dialogue menu and just pass in the relevant line number?
Here's what I've been trying to make so far, this compiles but the game throws an error during dialogue and won't display any options using this code.
I don't want to have to create a unique procedure for every single dialogue line I want to play, but I can't seem to figure out how to create a generic reply procedure and pass the line number at the same time.
Specifically
OPTION("debug reply1", 297);
is replacing
// gsay_option(54, "debug reply1", Nicole_Debug, 50);
to try and pass the line number into the wrapped function.
Any hints?
Here's what I've been trying to make so far, this compiles but the game throws an error during dialogue and won't display any options using this code.
Code:
variable string;
variable MSG_LINE_NMBR;
#define OPTION(string, y) \
MSG_LINE_NMBR := y; \
gsay_option(54, string, Nicole_Debug, 50)
procedure Nicole_Debug begin
gsay_reply(54, MSG_LINE_NMBR);
gsay_option(54, "debug menu", Nicole_Debug, 50);
end
procedure Nicole_Debug_A begin
gsay_reply(54, 297);
OPTION("debug reply1", 297);
// gsay_option(54, "debug reply1", Nicole_Debug, 50);
gsay_option(54, "165 Nic_22 Rippers", Nicole_Debug1, 50);
gsay_option(54, "310 Nic_95 Rippers3", Nicole_Debug3, 50);
gsay_option(54, "226 Nic_42 Rippers2", Nicole_Debug2, 50);
gsay_option(54, "311 Nic_97 Rippers4", Nicole_Debug4, 50);
gsay_option(54, "169 Nic_23 Charitable", Nicole_Debug5, 50);
gsay_option(54, "172 Nic_24 Careful" , Nicole_Debug6, 50);
gsay_option(54, "next", Nicole_Debug_B, 50);
gsay_option(54, "end dialogue", NicoleEnd, 50);
end
I don't want to have to create a unique procedure for every single dialogue line I want to play, but I can't seem to figure out how to create a generic reply procedure and pass the line number at the same time.
Specifically
OPTION("debug reply1", 297);
is replacing
// gsay_option(54, "debug reply1", Nicole_Debug, 50);
to try and pass the line number into the wrapped function.
Any hints?