fffffffff
First time out of the vault
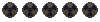
Hello I'm banana programmer and I've got huge problem.
I need to have timed_event_p_proc repeated over and over and i want it repeated for example 1 time per second so basically it sounds like:
And it looks like it works but when I save the game and load it back, it executes 2 times more, again and again save/load X time more. And when I exit the map and go back to my map it executes fine and beautiful again but this saving/loading game breaks everything.
I need to have timed_event_p_proc repeated over and over and i want it repeated for example 1 time per second so basically it sounds like:
Code:
procedure map_enter_p_proc begin
add_timer_event(self_obj,0,0);
end
procedure timed_event_p_proc begin
if (fixed_param==0) then begin
call repeatable_procedure;
add_timer_event(self_obj,game_ticks(1),0);
end
end
And it looks like it works but when I save the game and load it back, it executes 2 times more, again and again save/load X time more. And when I exit the map and go back to my map it executes fine and beautiful again but this saving/loading game breaks everything.